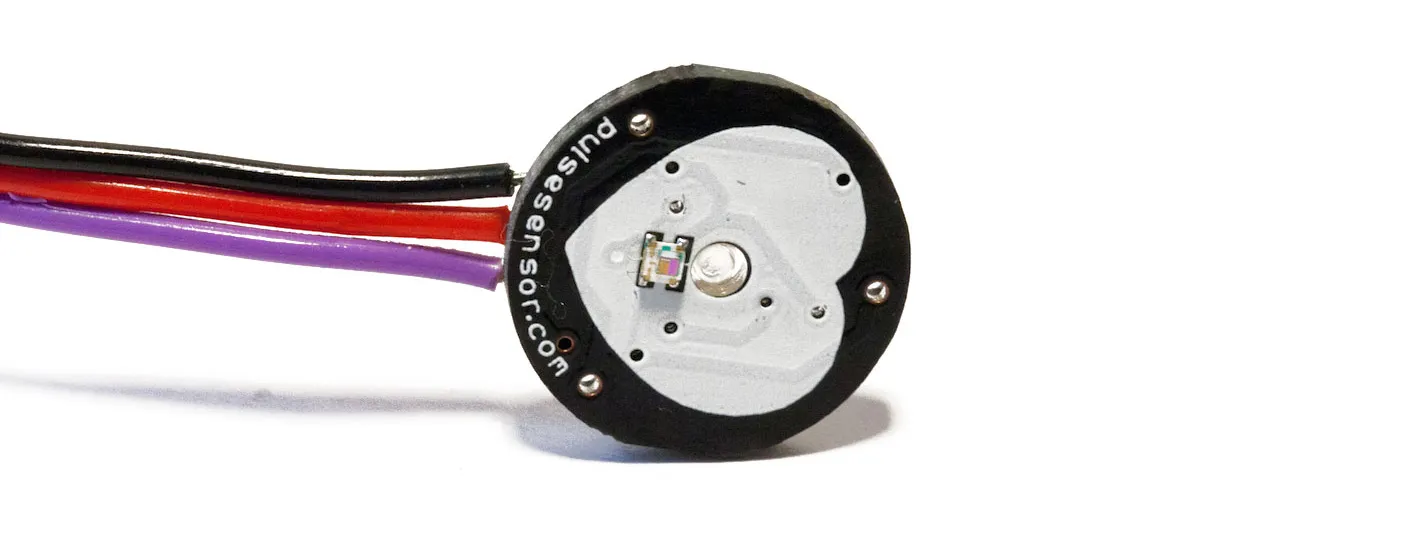
Make a Bluetooth Heart-Rate Sensor with Arduino
Measuring the heart rate is a very common project in healthcare, and it is for example quite easy to do with your smartphone for example. In this project, you are going to learn how to measure your heart rate with a pure open-source hardware solution based on Arduino.
We are going to see how to measure your heart rate using a dedicated Arduino-compatible sensor. Then, we will transmit this data via Bluetooth, and display it on your computer where it can be recorded. Let’s dive in!
Hardware & Software Requirements
We are first going to see what components are needed for this tutorial. First, you will need an Arduino Uno board. However, most Arduino boards will work for this tutorial.
You will also need the Pulse Rate Sensor Amped for Arduino. Other heart rate sensors could work as well, but the code of this article was made specifically for this model.
For the Bluetooth communication, we used an Adafruit EZ-Link Bluetooth module. Finally, you will also need a bunch of jumper cables and a breadboard to make all the necessary electrical connections.
This is the list of all the required components for this project:
On the software side, you will also need to download and install the Arduino aREST library.
Finally, you will need to download and install Node.js, that we will use for the final part when displaying data on your computer.
Hardware Configuration
We are now going to see how to assemble the project. First, take some cables to connect the 5V pin of the Arduino board to the red power rail on the breadboard, and GND of the Arduino board to the blue power rail on the breadboard.
After that, we are going to connect the pulse rate sensor. Simply connect the red cable to the red power rail, black cable to the blue power rail, and finally the remaining wire to analog pin A0 on the Arduino board.
For the Bluetooth module, place it first on the breadboard. Then, connect the power supply: connect the VIN pin to the red power rail, and connect the GND pin to the blue power rail. For now, don’t connect the TX and RX pins, as we need to program our Arduino board first.
Testing the Sensor
Now that our hardware is configured, we are going to write some test Arduino code to check that the heart rate sensor is working correctly. We first need to define a variable that will contain the heart beats per minute (BPM):
volatile int BPM;
Then, we define a bunch of variables that are needed for the heart rate detection algorithm to work:
volatile int Signal;
volatile int IBI = 600;
volatile boolean Pulse = false;
volatile boolean QS = false;
Now, in the setup() part of the sketch, we start the Serial communication, so we can display some data on the Arduino Serial monitor:
Serial.begin(115200);
We also call the interruptSetup function, which will initialise the algorithm used to find the heart rate (not detailed in this tutorial):
interruptSetup();
Now, in the loop() function of the sketch, if we detect a heart pulse (QS = 1), we print it on the Serial monitor:
// If heart beat is found
if (QS == true) {
// Print heart rate
Serial.print("Heart rate: ");
Serial.println(BPM);
// Reset the Quantified Self flag for next time
QS = false;
}
We repeat this operation every 20 milliseconds:
delay(20);
Note that all the code can be found inside the GitHub repository of the article:
https://github.com/openhomeautomation/bluetooth-pulse-rate-sensor
It’s now time to do a first test of our project. Upload the code to the Arduino board, and then open the Serial monitor. Then, put your finger on top of the heart rate sensor, making sure to tighten the band around the sensor so the tip of your finger is really in contact with the sensor. If needed, you can visit the sensor official help page.
You should see your heart rate being displayed inside the Serial monitor.
Displaying the Pulse Rate on Your Desktop
We are now going to build a simple way to display the heart rate on your desktop, inside a web app. To do so, we first need to modify the Arduino sketch. To help us, we will make use of the aREST library that will handle the communication via Bluetooth. It starts by including this library:
#include
We also declare a variable that will contain the measured BPM:
int measured_bpm;
In the setup() function of the sketch, we give a name to our project, and also expose the variable to the API so we can access it remotely:
// Give name and ID to device
rest.set_id("1");
rest.set_name("pulse_rate_sensor");
// Expose BPM to API
rest.variable("bpm",&measured_bpm);
In the loop() function, we store the measure BPM into our exposed variable:
measured_bpm = BPM;
We also handle the incoming data using the aREST library:
rest.handle(Serial);
This is basically it for the Arduino part. You can find the complete code at:
https://github.com/openhomeautomation/bluetooth-pulse-rate-sensor
You can now already upload the code to the Arduino board. Then, connect the RX & TX pins of the Bluetooth module. Connect TX to Arduino RX, and vice-versa. After that, pair the Bluetooth module with your computer using your computer Bluetooth settings.
We are now going to see how to use the application to display the data, based on Node.js. We won’t see all the details of this app, but basically once you download the file from the GitHub repository you just need to modify the Serial port corresponding to your Bluetooth module. This value can be found in your Arduino IDE under Tools>Port. Then, open the file called app.js, and modify the following line:
rest.addDevice('serial','/dev/tty.usbmodem1a12121', 115200);
Note that you can find the whole interface at:
https://github.com/openhomeautomation/bluetooth-pulse-rate-sensor
It’s now time to test the application. Go in the folder where you put all the application files, and type:
Then, start the application with:
Finally, go to your favorite web browser and type:
You should see the interface being displayed, with your heart rate being refreshed constantly.
How to Go Further
Let’s summarise what we did in this project. We built an open-source heart rate sensor based on Arduino, and connected it to your computer via Bluetooth. Therefore, you are now able to monitor your heart rate from your computer, just by putting your finger in the sensor.
You can improve this project by using the same concept to connect more healthcare sensors, for example a breath meter. You can also use the Bluetooth connection of the project to connect the heart rate sensor to a mobile application running on your phone or tablet.